77 KiB
Support Vector Machines¶
Exercise¶
Fraud in Wine¶
Wine fraud relates to the commercial aspects of wine. The most prevalent type of fraud is one where wines are adulterated, usually with the addition of cheaper products (e.g. juices) and sometimes with harmful chemicals and sweeteners (compensating for color or flavor).
Counterfeiting and the relabelling of inferior and cheaper wines to more expensive brands is another common type of wine fraud.
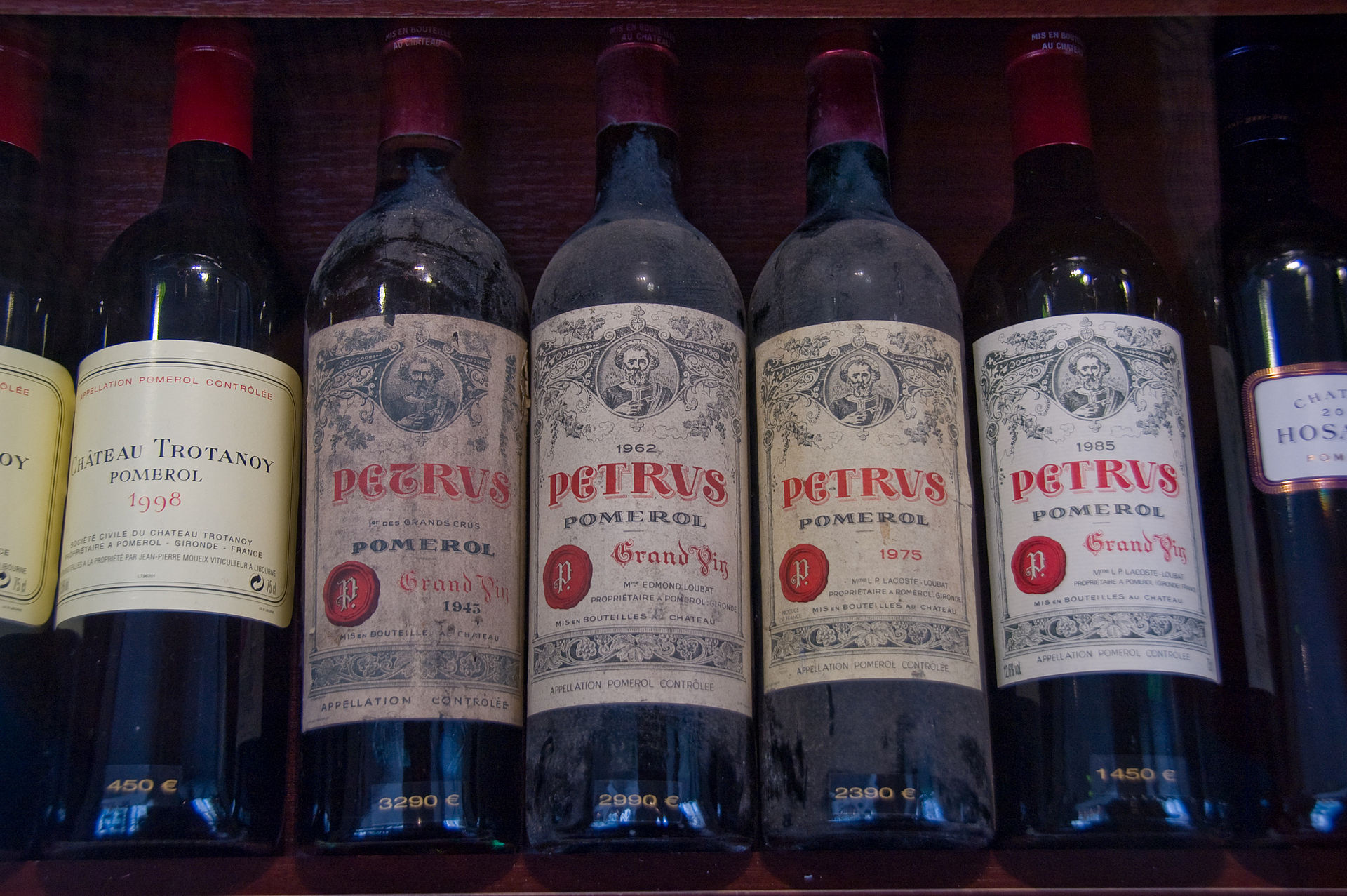
Project Goals¶
A distribution company that was recently a victim of fraud has completed an audit of various samples of wine through the use of chemical analysis on samples. The distribution company specializes in exporting extremely high quality, expensive wines, but was defrauded by a supplier who was attempting to pass off cheap, low quality wine as higher grade wine. The distribution company has hired you to attempt to create a machine learning model that can help detect low quality (a.k.a "fraud") wine samples. They want to know if it is even possible to detect such a difference.
Data Source: P. Cortez, A. Cerdeira, F. Almeida, T. Matos and J. Reis. Modeling wine preferences by data mining from physicochemical properties. In Decision Support Systems, Elsevier, 47(4):547-553, 2009.
TASK: Your overall goal is to use the wine dataset shown below to develop a machine learning model that attempts to predict if a wine is "Legit" or "Fraud" based on various chemical features. Complete the tasks below to follow along with the project.
Complete the Tasks in bold¶
TASK: Run the cells below to import the libraries and load the dataset.
import numpy as np
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
df = pd.read_csv("../DATA/wine_fraud.csv")
df.head()
TASK: What are the unique variables in the target column we are trying to predict (quality)?
TASK: Create a countplot that displays the count per category of Legit vs Fraud. Is the label/target balanced or unbalanced?
# CODE HERE
TASK: Let's find out if there is a difference between red and white wine when it comes to fraud. Create a countplot that has the wine type on the x axis with the hue separating columns by Fraud vs Legit.
# CODE HERE
TASK: What percentage of red wines are Fraud? What percentage of white wines are fraud?
TASK: Calculate the correlation between the various features and the "quality" column. To do this you may need to map the column to 0 and 1 instead of a string.
# CODE HERE
TASK: Create a bar plot of the correlation values to Fraudlent wine.
# CODE HERE
TASK: Create a clustermap with seaborn to explore the relationships between variables.
# CODE HERE
Machine Learning Model¶
TASK: Convert the categorical column "type" from a string or "red" or "white" to dummy variables:
# CODE HERE
TASK: Separate out the data into X features and y target label ("quality" column)
TASK: Perform a Train|Test split on the data, with a 10% test size. Note: The solution uses a random state of 101
TASK: Scale the X train and X test data.
TASK: Create an instance of a Support Vector Machine classifier. Previously we have left this model "blank", (e.g. with no parameters). However, we already know that the classes are unbalanced, in an attempt to help alleviate this issue, we can automatically adjust weights inversely proportional to class frequencies in the input data with a argument call in the SVC() call. Check out the documentation for SVC online and look up what the argument\parameter is.
# CODE HERE
TASK: Use a GridSearchCV to run a grid search for the best C and gamma parameters.
# CODE HERE
TASK: Display the confusion matrix and classification report for your model.
TASK: Finally, think about how well this model performed, would you suggest using it? Realistically will this work?
# ANSWER: View the solutions video for full discussion on this.